Thoughts on Component Architecture
Why is Component Architecture Important?
In frontend development, creating a clear separation of concerns within an application simplifies code management, improves code reusability, and enhances team collaboration. By structuring components thoughtfully, you can achieve:
- Code Reusability: Modular components allow easy reuse across different parts of the application.
- Improved Maintainability: Isolated concerns reduce complexity, making it easier to update, debug, and extend the code.
- Increased Development Speed: Having reusable components means less rebuilding, enabling faster feature development.
A well-architected component system ultimately makes applications more scalable and sustainable over time.
Smart Components vs. Presentation Components
Component-based architecture divides the application into smart components and presentation components:
-
Smart Components
- These components encapsulate business logic and manage data flow within the application.
- They typically fetch data, manage state, and dispatch updates to child components.
- Smart components are often at the top of the hierarchy, commonly used for pages, containers, or complex UI structures like tables.
Example: A
UserDashboard
component that fetches user data and passes it down to child components, likeUserProfile
orUserStats
. -
Presentation Components
- Presentation components are responsible for rendering UI and should contain minimal to no business logic.
- They are reusable building blocks that focus on layout and styling, allowing for consistent design patterns across the app.
- Ideally, these components are flexible and customizable without being overly granular.
Example: A
UserProfile
component that displays user information passed down from a parent component, formatted according to predefined templates.
Why Are Smart Components Important?
Smart components are essential because they encapsulate business logic, acting as the data controllers of the application. By handling data fetching, state management, and event handling, they ensure that presentation components can focus solely on display logic.
Benefits of Smart Components
- Encapsulated Data Flow: Data is managed in one place, making it easier to track and update as needed.
- Consistent State Management: Allows for predictable data flow, improving code readability and making debugging easier.
Why Are Presentation Components Important?
Presentation components provide reusable, standardized templates for the application's UI. They ensure that visual elements are isolated from business logic, fostering consistent styling and behavior across the application.
Benefits of Presentation Components
- Reusability: Encapsulated templates make it easy to reuse presentation logic, decreasing the amount of repetitive code.
- Maintainability: Presentation components allow for rapid UI adjustments across the app without impacting business logic.
Splitting the Application: Best Practices
Component-based architecture often starts by building everything within a smart component and segmenting out presentation components as the reusable aspects become clear. It’s common for beginners to over-engineer at this stage, but avoid creating unnecessary components too early, as this can add complexity without adding value.
Guidelines:
- Start Simple: Begin with fewer components and only split out when a pattern emerges.
- Refactor When Needed: It’s better to start with simpler structures and refactor as the application grows.
- Avoid Premature Optimization: Overly granular components can complicate maintenance. Aim for a balance between reusability and readability.
Example Implementation
Here’s an example illustrating how to structure smart and presentation components in a React application.
Smart Component (UserDashboard):
import React, { useState, useEffect } from 'react';
import UserProfile from './UserProfile';
function UserDashboard() {
const [user, setUser] = useState(null);
useEffect(() => {
// Fetch user data here
fetchUserData().then((data) => setUser(data));
}, []);
return <>{user && <UserProfile user={user} />}</>;
}
export default UserDashboard;
Presentation Component (UserProfile):
import React from 'react';
function UserProfile({ user }) {
return (
<div>
<h2>{user.name}</h2>
<p>{user.email}</p>
</div>
);
}
export default UserProfile;
In this example, UserDashboard
is responsible for fetching and managing user data, while UserProfile
focuses solely on presenting that data. This separation ensures a clear distinction between business and presentation logic.
Final Thoughts
Component architecture is an evolving process that becomes more intuitive with experience. Focus on functionality, then refactor as patterns emerge to avoid over-engineering.
Key Takeaways:
- Separate business and presentation logic to promote reusability and maintainability.
- Start with simpler structures, adding complexity only as needed.
- Refactor thoughtfully as the application grows to enhance readability and performance.
Next Steps
To deepen your understanding:
- Experiment with component architecture in a small project.
- Study advanced component patterns, such as higher-order components and render props.
- Explore resources like React’s component documentation for more insights.
With these practices, you’ll develop a strong foundation in building scalable, maintainable frontend applications.
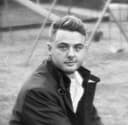
Written by Roman Khrystynych who lives and works in Toronto building interesting things.